模块化可以帮助我们组织代码,提高代码的可维护性和复用性。
什么是模块化
模块化将代码分割成独立的、可复用的模块,每个模块只负责一个特定的功能。
这个概念类似前面讲过的函数。但模块化通常是指的是某个大的功能,而不只是一个小的方法。
所以模块化的优势包括:
模块化规范
JavaScript 中常见的模块化规范有 CommonJS、AMD、UMD 和 ES6 模块。
CommonJS
CommonJS 主要用于 Node.js 环境。
它使用 require
导入模块,使用 module.exports
导出模块。
比如,我们有一个数学计算模块,里面暂时只有 add
这一个方法。
// 数学模块代码 math.js,这里只写了加法方法。 module.exports.add = function (a, b) { return a + b; }; // 在main.js中使用数学模块时,使用require导入math.js模块 const math = require('./math'); console.log(math.add(2, 3)); // 输出: 5
AMD(Asynchronous Module Definition)
AMD 主要用于浏览器环境,使用 define
定义模块,使用 require
导入模块。
// math.js define([], function () { return { add: function (a, b) { return a + b; }, }; }); // main.js require(['./math'], function (math) { console.log(math.add(2, 3)); // 输出: 5 });
这里的模块实现和使用和前面一样,只是使用了 AMD 规范。
UMD(Universal Module Definition)
UMD 则是兼容了 CommonJS 和 AMD,适用于需要兼容多种模块化规范的场景。
代码看起来有点复杂,暂时不明白也没有关系,知道 UMD 这个规划的概念就好。
(function (root, factory) { if (typeof define === 'function' && define.amd) { // AMD define([], factory); } else if (typeof module === 'object' && module.exports) { // CommonJS module.exports = factory(); } else { // Browser globals root.math = factory(); } })(this, function () { return { add: function (a, b) { return a + b; }, }; });
ES6 模块
ES6 模块是现代浏览器和 Node.js 的标准,它使用 import
和 export
关键字。
// math.js export function add(a, b) { return a + b; } // main.js import { add } from './math.js'; console.log(add(2, 3)); // 输出: 5
模块打包工具
在浏览器中使用模块化代码,需要使用模块打包工具,如 Webpack、Rollup 和 Parcel。
Webpack
Webpack 的配置文件通常命名为 webpack.config.js
,它是一个导出配置对象的 JavaScript 文件。
const path = require('path'); const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'], }, { test: /\.(png|svg|jpg|jpeg|gif)$/i, type: 'asset/resource', }, ], }, plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), ], devServer: { contentBase: './dist', hot: true, }, };
基于上述例子,依次来看看。
entry
是 Webpack 构建的起点,指示 Webpack 从哪个文件开始构建依赖图。
output
配置 Webpack 如何以及在哪里输出打包后的文件。
加载器用于转换模块的源代码。
Webpack 本身只能理解 JavaScript 和 JSON 文件,通过加载器可以处理其他类型的文件(如 CSS、图片、TypeScript 等)。
插件用于执行范围更广的任务,如打包优化、资源管理、环境变量注入等。
所以 Webpack 将所有类型的资源(JavaScript、CSS、图片等)视为模块,通过依赖图将它们打包成一个或多个 bundle。
热模块 hot:true
替换允许在应用程序运行时替换、添加或删除模块,而无需重新加载整个页面。
代码拆分可以将代码分割成不同的 bundle,以便按需加载,提高性能。
// webpack.config.js module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, };
Rollup
Rollup 是一个专注于 ES6 模块的打包工具,生成的包体积较小。
// rollup.config.js export default { input: 'src/main.js', output: { file: 'dist/bundle.js', format: 'iife', }, };
Parcel
Parcel 是一个零配置的打包工具,使用简单,旨在简化开发者的工作流程。
parcel build src/index.html
动态导入模块
ES6 模块支持动态导入,可以在需要时加载模块,提高性能。
// main.js document.getElementById('loadButton').addEventListener('click', async () => { const { add } = await import('./math.js'); console.log(add(2, 3)); // 输出: 5 });
模块化最佳实践
示例:网页开关
来看一个使用模块化实现网页开关功能的示例:
// toggle.js export function toggleVisibility(element) { if (element.style.display === 'none') { element.style.display = 'block'; } else { element.style.display = 'none'; } } // main.js import { toggleVisibility } from './toggle.js'; document.getElementById('toggleButton').addEventListener('click', () => { const content = document.getElementById('content'); toggleVisibility(content); });
HTML 部分:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Toggle Example</title> </head> <body> <button id="toggleButton">开关</button> <div id="content">这里是内容</div> <script type="module" src="014-main.js"></script> </body> </html>
看一下效果
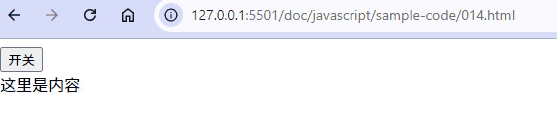
总结
🍑 模块化将代码分割成独立的、可复用的模块,每个模块只负责一个特定的功能。
🍑 JavaScript 中常见的模块化规范有 CommonJS、AMD、UMD 和 ES6 模块。
🍑 模块打包工具有 Webpack、Rollup 和 Parcel。
该文章在 2024/10/29 9:00:02 编辑过